Demonstration of API using OpenWeatherMap.org#
This demonstration is heavily inspired by NeuralNine’s video.
You need a free account from here.
The VS Code extension JSON viewer is recommended for viewing downloaded JSON content.
Set your maximum API calls to 1000 per day to make sure you are under the limit for billing.
To run the examples, download your API key, save it in the right folder (see below) in a file called api_key_OpenWeather, containing only the key (no spaces or “enters”).
# Imports
import datetime as dt
import requests
import json
Current weather#
Common definitions to use for all requests#
BASE_URL = "http://api.openweathermap.org/data/2.5/weather?"
API_KEY = open('../../../No_sync/api_key_OpenWeather','r').read()
CITY = "Ski"
url = BASE_URL + "q=" + CITY + "&appid=" + API_KEY
Request current weather in chosen city#
response = requests.get(url).json()
print(response)
{'coord': {'lon': 10.8358, 'lat': 59.7195}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'base': 'stations', 'main': {'temp': 269.33, 'feels_like': 269.33, 'temp_min': 269.14, 'temp_max': 270.72, 'pressure': 1029, 'humidity': 96, 'sea_level': 1029, 'grnd_level': 1012}, 'visibility': 10000, 'wind': {'speed': 1.26, 'deg': 98, 'gust': 3.47}, 'clouds': {'all': 100}, 'dt': 1733343751, 'sys': {'type': 2, 'id': 2006772, 'country': 'NO', 'sunrise': 1733298944, 'sunset': 1733321916}, 'timezone': 3600, 'id': 3139081, 'name': 'Ski', 'cod': 200}
# Write JSON to file for viewing
with open('downloads/weather.json', 'w') as f:
json.dump(response, f, indent=4)
Conversion functions#
Changing scales can make results more interpretable
# Kelvin to Celsius
def kelvin_to_celsius(temp):
return temp - 273.15
# Meters per second to knots
def mps_to_knots(speed):
return speed * 1.943844
Print some weather properties#
# Current temperature
temp_kelvin = response['main']['temp']
temp_celsius = kelvin_to_celsius(temp_kelvin)
print(f"The current temperature in {CITY} is {temp_celsius:.1f} °C")
The current temperature in Ski is -3.8 °C
# Sunrise and sunset today in local time
sunrise = dt.datetime.fromtimestamp(response['sys']['sunrise'])
sunset = dt.datetime.fromtimestamp(response['sys']['sunset'])
print(f"Sunrise today is at {sunrise:%H:%M} and sunset is at {sunset:%H:%M}")
Sunrise today is at 08:55 and sunset is at 15:18
# Wind direction and speed
wind_knots = mps_to_knots(response['wind']['speed'])
print(f"Wind today is from {response['wind']['deg']}° at {round(wind_knots,1)} knots")
Wind today is from 98° at 2.4 knots
Forecasted weather#
Common definitions to use for all requests#
BASE_URL = "https://api.openweathermap.org/data/2.5/forecast?"
CITY = "Mo i Rana"
urlF = BASE_URL + "q=" + CITY + "&appid=" + API_KEY
Request forecasted weather in chosen city#
responseF = requests.get(urlF).json()
#print(json.dumps(responseF, indent=4))
print(responseF)
{'cod': '200', 'message': 0, 'cnt': 40, 'list': [{'dt': 1733346000, 'main': {'temp': 263.41, 'feels_like': 263.41, 'temp_min': 263.41, 'temp_max': 263.64, 'pressure': 1022, 'sea_level': 1022, 'grnd_level': 967, 'humidity': 92, 'temp_kf': -0.23}, 'weather': [{'id': 800, 'main': 'Clear', 'description': 'clear sky', 'icon': '01n'}], 'clouds': {'all': 0}, 'wind': {'speed': 0.41, 'deg': 62, 'gust': 0.3}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-04 21:00:00'}, {'dt': 1733356800, 'main': {'temp': 263.9, 'feels_like': 263.9, 'temp_min': 263.9, 'temp_max': 264.88, 'pressure': 1022, 'sea_level': 1022, 'grnd_level': 967, 'humidity': 90, 'temp_kf': -0.98}, 'weather': [{'id': 802, 'main': 'Clouds', 'description': 'scattered clouds', 'icon': '03n'}], 'clouds': {'all': 33}, 'wind': {'speed': 0.89, 'deg': 58, 'gust': 0.29}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-05 00:00:00'}, {'dt': 1733367600, 'main': {'temp': 264.11, 'feels_like': 259.77, 'temp_min': 264.11, 'temp_max': 264.46, 'pressure': 1021, 'sea_level': 1021, 'grnd_level': 965, 'humidity': 87, 'temp_kf': -0.35}, 'weather': [{'id': 803, 'main': 'Clouds', 'description': 'broken clouds', 'icon': '04n'}], 'clouds': {'all': 66}, 'wind': {'speed': 2.25, 'deg': 71, 'gust': 1.68}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-05 03:00:00'}, {'dt': 1733378400, 'main': {'temp': 262.73, 'feels_like': 258.11, 'temp_min': 262.73, 'temp_max': 262.73, 'pressure': 1018, 'sea_level': 1018, 'grnd_level': 963, 'humidity': 84, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 96}, 'wind': {'speed': 2.28, 'deg': 73, 'gust': 1.61}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-05 06:00:00'}, {'dt': 1733389200, 'main': {'temp': 264.61, 'feels_like': 259.88, 'temp_min': 264.61, 'temp_max': 264.61, 'pressure': 1016, 'sea_level': 1016, 'grnd_level': 961, 'humidity': 84, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 2.6, 'deg': 72, 'gust': 1.61}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-05 09:00:00'}, {'dt': 1733400000, 'main': {'temp': 264.11, 'feels_like': 258.23, 'temp_min': 264.11, 'temp_max': 264.11, 'pressure': 1014, 'sea_level': 1014, 'grnd_level': 960, 'humidity': 85, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04d'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.51, 'deg': 79, 'gust': 3.04}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'd'}, 'dt_txt': '2024-12-05 12:00:00'}, {'dt': 1733410800, 'main': {'temp': 264.32, 'feels_like': 258.51, 'temp_min': 264.32, 'temp_max': 264.32, 'pressure': 1012, 'sea_level': 1012, 'grnd_level': 958, 'humidity': 86, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.49, 'deg': 79, 'gust': 3.55}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-05 15:00:00'}, {'dt': 1733421600, 'main': {'temp': 263.62, 'feels_like': 257.55, 'temp_min': 263.62, 'temp_max': 263.62, 'pressure': 1011, 'sea_level': 1011, 'grnd_level': 956, 'humidity': 86, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.6, 'deg': 77, 'gust': 3.17}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-05 18:00:00'}, {'dt': 1733432400, 'main': {'temp': 264.26, 'feels_like': 258.04, 'temp_min': 264.26, 'temp_max': 264.26, 'pressure': 1008, 'sea_level': 1008, 'grnd_level': 954, 'humidity': 86, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.9, 'deg': 82, 'gust': 3.47}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-05 21:00:00'}, {'dt': 1733443200, 'main': {'temp': 264.44, 'feels_like': 258.58, 'temp_min': 264.44, 'temp_max': 264.44, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 953, 'humidity': 86, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.57, 'deg': 84, 'gust': 3.2}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-06 00:00:00'}, {'dt': 1733454000, 'main': {'temp': 264.54, 'feels_like': 259.19, 'temp_min': 264.54, 'temp_max': 264.54, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 953, 'humidity': 88, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.1, 'deg': 78, 'gust': 2.47}, 'visibility': 10000, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-06 03:00:00'}, {'dt': 1733464800, 'main': {'temp': 268.48, 'feels_like': 265.48, 'temp_min': 268.48, 'temp_max': 268.48, 'pressure': 1006, 'sea_level': 1006, 'grnd_level': 953, 'humidity': 88, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.85, 'deg': 76, 'gust': 1.77}, 'visibility': 9453, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-06 06:00:00'}, {'dt': 1733475600, 'main': {'temp': 269.39, 'feels_like': 267.27, 'temp_min': 269.39, 'temp_max': 269.39, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 953, 'humidity': 93, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.41, 'deg': 66, 'gust': 0.86}, 'visibility': 301, 'pop': 0.34, 'snow': {'3h': 0.18}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-06 09:00:00'}, {'dt': 1733486400, 'main': {'temp': 270.07, 'feels_like': 268.06, 'temp_min': 270.07, 'temp_max': 270.07, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 954, 'humidity': 94, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13d'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.4, 'deg': 86, 'gust': 1.24}, 'visibility': 1084, 'pop': 0.28, 'snow': {'3h': 0.2}, 'sys': {'pod': 'd'}, 'dt_txt': '2024-12-06 12:00:00'}, {'dt': 1733497200, 'main': {'temp': 269.66, 'feels_like': 266.3, 'temp_min': 269.66, 'temp_max': 269.66, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 954, 'humidity': 93, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 98}, 'wind': {'speed': 2.25, 'deg': 86, 'gust': 2.06}, 'pop': 0.5, 'snow': {'3h': 0.25}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-06 15:00:00'}, {'dt': 1733508000, 'main': {'temp': 269.18, 'feels_like': 266.51, 'temp_min': 269.18, 'temp_max': 269.18, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 954, 'humidity': 89, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 99}, 'wind': {'speed': 1.71, 'deg': 60, 'gust': 0.79}, 'visibility': 10000, 'pop': 0.1, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-06 18:00:00'}, {'dt': 1733518800, 'main': {'temp': 268.72, 'feels_like': 268.72, 'temp_min': 268.72, 'temp_max': 268.72, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 954, 'humidity': 91, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.02, 'deg': 324, 'gust': 0.13}, 'visibility': 9213, 'pop': 0, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-06 21:00:00'}, {'dt': 1733529600, 'main': {'temp': 271.12, 'feels_like': 271.12, 'temp_min': 271.12, 'temp_max': 271.12, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 954, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.14, 'deg': 78, 'gust': 0.84}, 'pop': 1, 'snow': {'3h': 1.58}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-07 00:00:00'}, {'dt': 1733540400, 'main': {'temp': 270.1, 'feels_like': 270.1, 'temp_min': 270.1, 'temp_max': 270.1, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 954, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 0.85, 'deg': 66, 'gust': 0.42}, 'visibility': 8, 'pop': 0.97, 'snow': {'3h': 0.45}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-07 03:00:00'}, {'dt': 1733551200, 'main': {'temp': 271.28, 'feels_like': 271.28, 'temp_min': 271.28, 'temp_max': 271.28, 'pressure': 1007, 'sea_level': 1007, 'grnd_level': 954, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 0.7, 'deg': 46, 'gust': 0.08}, 'pop': 1, 'snow': {'3h': 1.41}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-07 06:00:00'}, {'dt': 1733562000, 'main': {'temp': 271.5, 'feels_like': 271.5, 'temp_min': 271.5, 'temp_max': 271.5, 'pressure': 1008, 'sea_level': 1008, 'grnd_level': 955, 'humidity': 93, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.17, 'deg': 59, 'gust': 0.57}, 'visibility': 4800, 'pop': 0.61, 'snow': {'3h': 0.31}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-07 09:00:00'}, {'dt': 1733572800, 'main': {'temp': 266.71, 'feels_like': 263.84, 'temp_min': 266.71, 'temp_max': 266.71, 'pressure': 1010, 'sea_level': 1010, 'grnd_level': 956, 'humidity': 93, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04d'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.61, 'deg': 76, 'gust': 1.33}, 'visibility': 10000, 'pop': 0.08, 'sys': {'pod': 'd'}, 'dt_txt': '2024-12-07 12:00:00'}, {'dt': 1733583600, 'main': {'temp': 269.59, 'feels_like': 269.59, 'temp_min': 269.59, 'temp_max': 269.59, 'pressure': 1010, 'sea_level': 1010, 'grnd_level': 957, 'humidity': 93, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 99}, 'wind': {'speed': 1.02, 'deg': 58, 'gust': 0.51}, 'visibility': 981, 'pop': 0.26, 'snow': {'3h': 0.11}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-07 15:00:00'}, {'dt': 1733594400, 'main': {'temp': 268.1, 'feels_like': 268.1, 'temp_min': 268.1, 'temp_max': 268.1, 'pressure': 1011, 'sea_level': 1011, 'grnd_level': 958, 'humidity': 93, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 99}, 'wind': {'speed': 0.4, 'deg': 72, 'gust': 0.16}, 'visibility': 9420, 'pop': 0.36, 'snow': {'3h': 0.27}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-07 18:00:00'}, {'dt': 1733605200, 'main': {'temp': 268.65, 'feels_like': 268.65, 'temp_min': 268.65, 'temp_max': 268.65, 'pressure': 1012, 'sea_level': 1012, 'grnd_level': 959, 'humidity': 91, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 0.59, 'deg': 123, 'gust': 0.61}, 'pop': 0.54, 'snow': {'3h': 0.21}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-07 21:00:00'}, {'dt': 1733616000, 'main': {'temp': 270.67, 'feels_like': 270.67, 'temp_min': 270.67, 'temp_max': 270.67, 'pressure': 1013, 'sea_level': 1013, 'grnd_level': 960, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 0.77, 'deg': 260, 'gust': 0.82}, 'pop': 1, 'snow': {'3h': 1.56}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-08 00:00:00'}, {'dt': 1733626800, 'main': {'temp': 271.64, 'feels_like': 271.64, 'temp_min': 271.64, 'temp_max': 271.64, 'pressure': 1012, 'sea_level': 1012, 'grnd_level': 959, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 0.66, 'deg': 200, 'gust': 0.5}, 'visibility': 652, 'pop': 1, 'snow': {'3h': 0.92}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-08 03:00:00'}, {'dt': 1733637600, 'main': {'temp': 272.99, 'feels_like': 272.99, 'temp_min': 272.99, 'temp_max': 272.99, 'pressure': 1013, 'sea_level': 1013, 'grnd_level': 961, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 1.32, 'deg': 209, 'gust': 1.59}, 'visibility': 108, 'pop': 1, 'snow': {'3h': 5.33}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-08 06:00:00'}, {'dt': 1733648400, 'main': {'temp': 274.84, 'feels_like': 271.87, 'temp_min': 274.84, 'temp_max': 274.84, 'pressure': 1016, 'sea_level': 1016, 'grnd_level': 963, 'humidity': 99, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 2.78, 'deg': 237, 'gust': 4.7}, 'visibility': 92, 'pop': 1, 'snow': {'3h': 2.92}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-08 09:00:00'}, {'dt': 1733659200, 'main': {'temp': 276.13, 'feels_like': 272, 'temp_min': 276.13, 'temp_max': 276.13, 'pressure': 1017, 'sea_level': 1017, 'grnd_level': 965, 'humidity': 98, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13d'}], 'clouds': {'all': 100}, 'wind': {'speed': 4.93, 'deg': 235, 'gust': 9.44}, 'visibility': 44, 'pop': 1, 'snow': {'3h': 1.83}, 'sys': {'pod': 'd'}, 'dt_txt': '2024-12-08 12:00:00'}, {'dt': 1733670000, 'main': {'temp': 276.15, 'feels_like': 272.83, 'temp_min': 276.15, 'temp_max': 276.15, 'pressure': 1019, 'sea_level': 1019, 'grnd_level': 967, 'humidity': 99, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.57, 'deg': 224, 'gust': 5.84}, 'visibility': 210, 'pop': 1, 'snow': {'3h': 3.73}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-08 15:00:00'}, {'dt': 1733680800, 'main': {'temp': 276.14, 'feels_like': 272.88, 'temp_min': 276.14, 'temp_max': 276.14, 'pressure': 1023, 'sea_level': 1023, 'grnd_level': 970, 'humidity': 99, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.49, 'deg': 256, 'gust': 4.73}, 'visibility': 28, 'pop': 1, 'snow': {'3h': 5.21}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-08 18:00:00'}, {'dt': 1733691600, 'main': {'temp': 276.05, 'feels_like': 273.03, 'temp_min': 276.05, 'temp_max': 276.05, 'pressure': 1027, 'sea_level': 1027, 'grnd_level': 974, 'humidity': 99, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.13, 'deg': 257, 'gust': 4.47}, 'visibility': 28, 'pop': 1, 'snow': {'3h': 1.74}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-08 21:00:00'}, {'dt': 1733702400, 'main': {'temp': 276, 'feels_like': 272.19, 'temp_min': 276, 'temp_max': 276, 'pressure': 1031, 'sea_level': 1031, 'grnd_level': 977, 'humidity': 98, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 4.29, 'deg': 261, 'gust': 5.07}, 'visibility': 164, 'pop': 1, 'snow': {'3h': 0.69}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-09 00:00:00'}, {'dt': 1733713200, 'main': {'temp': 272.68, 'feels_like': 269.06, 'temp_min': 272.68, 'temp_max': 272.68, 'pressure': 1034, 'sea_level': 1034, 'grnd_level': 980, 'humidity': 98, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 100}, 'wind': {'speed': 3.03, 'deg': 263, 'gust': 3.78}, 'visibility': 1636, 'pop': 0.26, 'snow': {'3h': 0.21}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-09 03:00:00'}, {'dt': 1733724000, 'main': {'temp': 270.86, 'feels_like': 267.69, 'temp_min': 270.86, 'temp_max': 270.86, 'pressure': 1036, 'sea_level': 1036, 'grnd_level': 981, 'humidity': 95, 'temp_kf': 0}, 'weather': [{'id': 804, 'main': 'Clouds', 'description': 'overcast clouds', 'icon': '04n'}], 'clouds': {'all': 99}, 'wind': {'speed': 2.26, 'deg': 254, 'gust': 3.15}, 'visibility': 8003, 'pop': 0.01, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-09 06:00:00'}, {'dt': 1733734800, 'main': {'temp': 270.64, 'feels_like': 268.58, 'temp_min': 270.64, 'temp_max': 270.64, 'pressure': 1037, 'sea_level': 1037, 'grnd_level': 982, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13n'}], 'clouds': {'all': 99}, 'wind': {'speed': 1.47, 'deg': 222, 'gust': 1.53}, 'visibility': 234, 'pop': 0.9, 'snow': {'3h': 0.42}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-09 09:00:00'}, {'dt': 1733745600, 'main': {'temp': 273.62, 'feels_like': 270.39, 'temp_min': 273.62, 'temp_max': 273.62, 'pressure': 1035, 'sea_level': 1035, 'grnd_level': 981, 'humidity': 97, 'temp_kf': 0}, 'weather': [{'id': 600, 'main': 'Snow', 'description': 'light snow', 'icon': '13d'}], 'clouds': {'all': 99}, 'wind': {'speed': 2.8, 'deg': 219, 'gust': 3.31}, 'visibility': 242, 'pop': 1, 'snow': {'3h': 1.49}, 'sys': {'pod': 'd'}, 'dt_txt': '2024-12-09 12:00:00'}, {'dt': 1733756400, 'main': {'temp': 275.22, 'feels_like': 272.16, 'temp_min': 275.22, 'temp_max': 275.22, 'pressure': 1033, 'sea_level': 1033, 'grnd_level': 980, 'humidity': 99, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 99}, 'wind': {'speed': 2.97, 'deg': 222, 'gust': 4.54}, 'visibility': 55, 'pop': 1, 'snow': {'3h': 3.03}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-09 15:00:00'}, {'dt': 1733767200, 'main': {'temp': 276.45, 'feels_like': 273.07, 'temp_min': 276.45, 'temp_max': 276.45, 'pressure': 1033, 'sea_level': 1033, 'grnd_level': 980, 'humidity': 99, 'temp_kf': 0}, 'weather': [{'id': 601, 'main': 'Snow', 'description': 'snow', 'icon': '13n'}], 'clouds': {'all': 99}, 'wind': {'speed': 3.77, 'deg': 230, 'gust': 6.35}, 'visibility': 35, 'pop': 1, 'snow': {'3h': 1.77}, 'sys': {'pod': 'n'}, 'dt_txt': '2024-12-09 18:00:00'}], 'city': {'id': 3145614, 'name': 'Mo i Rana', 'coord': {'lat': 66.3128, 'lon': 14.1428}, 'country': 'NO', 'population': 17853, 'timezone': 3600, 'sunrise': 1733303288, 'sunset': 1733315984}}
# Write JSON to file for viewing
with open('downloads/forecast.json', 'w') as f:
json.dump(responseF, f, indent=4)
When and what?#
Check contents and time stamps
# Content of responseF
responseF.keys()
dict_keys(['cod', 'message', 'cnt', 'list', 'city'])
# Number of forecasts
print(len(responseF["list"]))
40
# Print forecast times
for forecast in responseF["list"]:
print(forecast["dt_txt"])
2024-12-04 21:00:00
2024-12-05 00:00:00
2024-12-05 03:00:00
2024-12-05 06:00:00
2024-12-05 09:00:00
2024-12-05 12:00:00
2024-12-05 15:00:00
2024-12-05 18:00:00
2024-12-05 21:00:00
2024-12-06 00:00:00
2024-12-06 03:00:00
2024-12-06 06:00:00
2024-12-06 09:00:00
2024-12-06 12:00:00
2024-12-06 15:00:00
2024-12-06 18:00:00
2024-12-06 21:00:00
2024-12-07 00:00:00
2024-12-07 03:00:00
2024-12-07 06:00:00
2024-12-07 09:00:00
2024-12-07 12:00:00
2024-12-07 15:00:00
2024-12-07 18:00:00
2024-12-07 21:00:00
2024-12-08 00:00:00
2024-12-08 03:00:00
2024-12-08 06:00:00
2024-12-08 09:00:00
2024-12-08 12:00:00
2024-12-08 15:00:00
2024-12-08 18:00:00
2024-12-08 21:00:00
2024-12-09 00:00:00
2024-12-09 03:00:00
2024-12-09 06:00:00
2024-12-09 09:00:00
2024-12-09 12:00:00
2024-12-09 15:00:00
2024-12-09 18:00:00
Make plots of omnipresent measurements and events#
We will later look at missing data, data only sporadically appearing and so on.
# Air pressure per period
pressures = []
timestamps = []
for forecast in responseF["list"]:
pressures.append(forecast["main"]["pressure"])
timestamps.append(dt.datetime.fromtimestamp(forecast["dt"]))
import matplotlib.pyplot as plt
plt.bar(timestamps, pressures)
plt.xticks(rotation=45)
plt.ylim(980, 1050)
plt.grid()
plt.ylabel("Air pressure (hPa)")
plt.title(f"Forecasted air pressure in {CITY}")
plt.show()
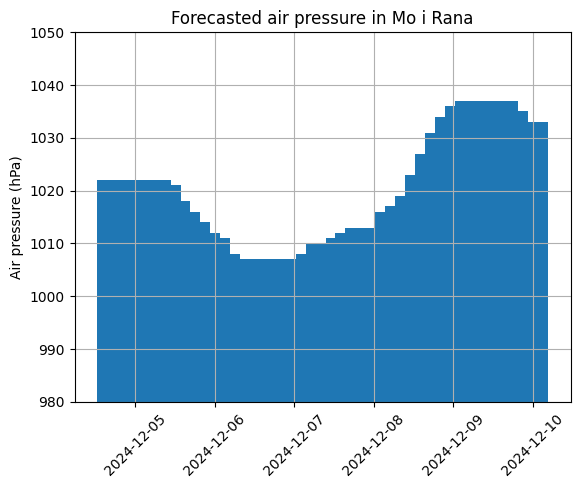
Exercise#
Make a new forecast request for your own hometown. Call your response something else than responseF.
If available, plot the humidity like we did with air pressure.
Precipitation#
… comes in two main flavours: rain and snow.
We need to check which is present and set to zero if it is abscent.
rain = []
snow = []
for forecast in responseF["list"]:
try: # Check if rain is present in forecast
rain.append(forecast["rain"]["3h"])
except KeyError:
rain.append(0)
try: # Check if snow is present in forecast
snow.append(forecast["snow"]["3h"])
except KeyError:
snow.append(0)
# Stacked bar chart with rain and snow
plt.bar(timestamps, rain, label="Rain")
plt.bar(timestamps, snow, label="Snow")
plt.xticks(rotation=45)
plt.grid()
plt.ylabel("Precipitation (mm)")
plt.title(f"Forecasted precipitation in {CITY}")
plt.legend()
plt.show()
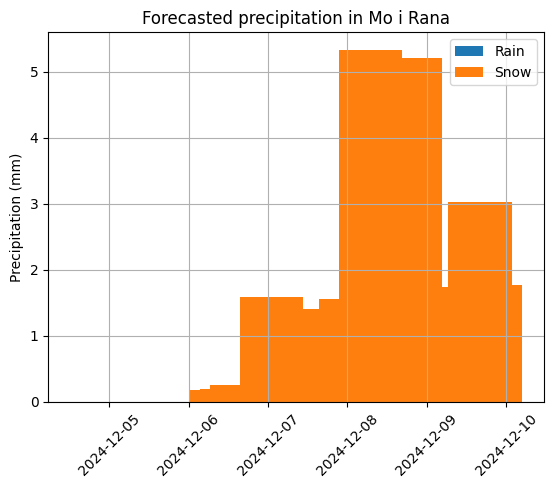