MongoDB#
To set up a MongoDB cluster, follow these steps:
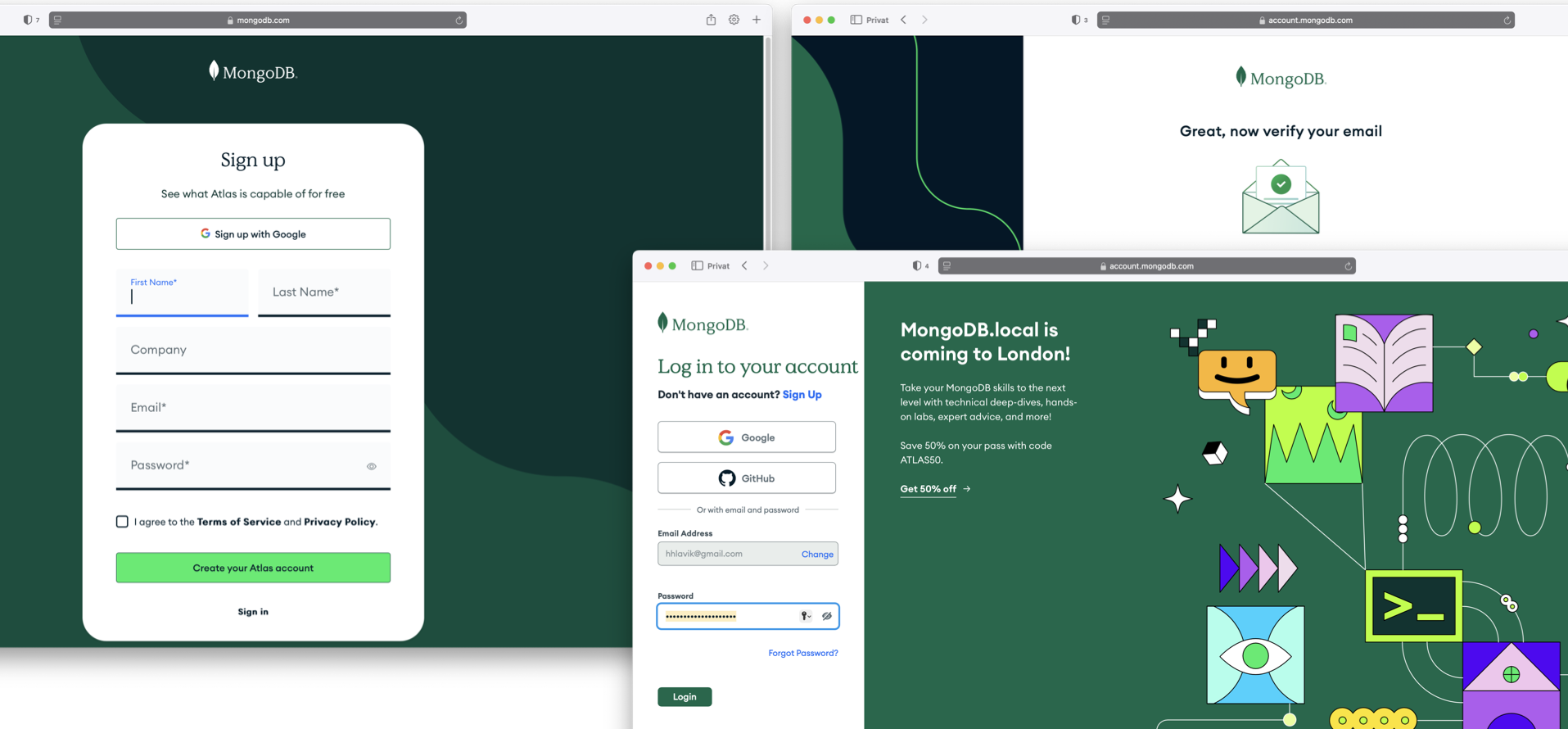
Create a free user#
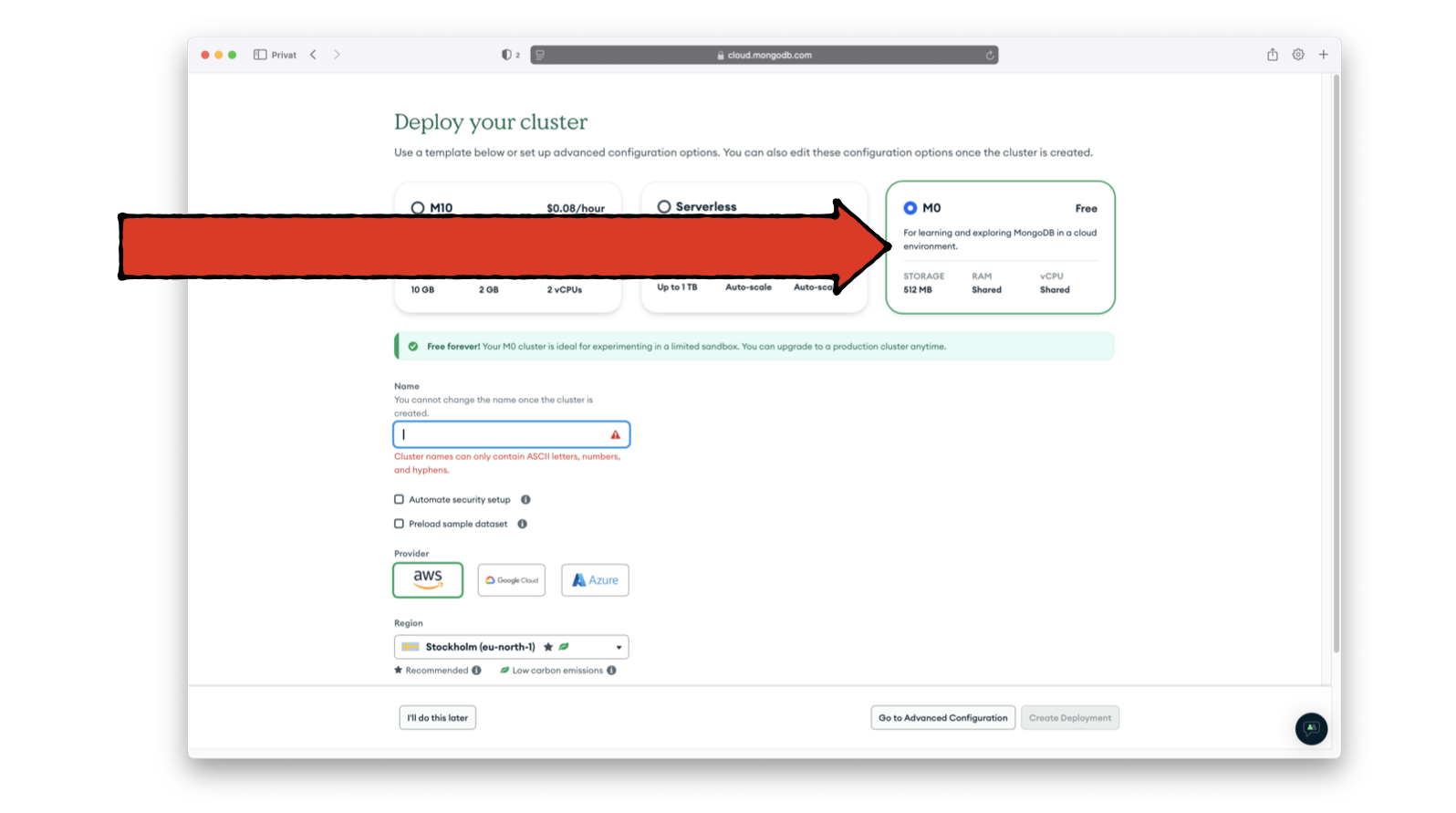
Select a username and password to be used through Python#
Open access for connection#
Add IP address 0.0.0.0/0 to avoid connection issues, then “Finish and close” at the bottom.
Choose connection type#
From the Overview menu, select Python as Application Development and click “Get connection string”
Follow the instructions (make sure to install from/in the correct Python environment).
“View full code example” for test code
MongoDB’s suggested code for checking if database is reachable#
NMBU’s VPN service seems to block a port related to MongoDB, thus needing to be disabled before running.
from pymongo.mongo_client import MongoClient
from pymongo.server_api import ServerApi
USR, PWD = open('../../../No_sync/MongoDB').read().splitlines()
uri = "mongodb+srv://khliland:"+PWD+"@ind320.rhc2o.mongodb.net/?retryWrites=true&w=majority&appName=IND320"
# Create a new client and connect to the server
client = MongoClient(uri, server_api=ServerApi('1'))
# Send a ping to confirm a successful connection
try:
client.admin.command('ping')
print("Pinged your deployment. You successfully connected to MongoDB!")
except Exception as e:
print(e)
Pinged your deployment. You successfully connected to MongoDB!
Connecting#
from pymongo.mongo_client import MongoClient
# Find the URI for your MongoDB cluster in the MongoDB dashboard:
# `Connect` -> `Drivers` -> Under heading 3.
uri = ("mongodb+srv://{}:{}@ind320.rhc2o.mongodb.net/"
"?retryWrites=true&w=majority&appName=IND320")
# Connecting to MongoDB with the chosen username and password.
USR, PWD = open('../../../No_sync/MongoDB').read().splitlines()
client = MongoClient(uri.format(USR, PWD))
# Selecting a database and a collection.
database = client['example']
collection = database['data']
Inserting#
The MongoDB structure is such that each database contains collections.
These collections contain documents, which are similar to dictionaries.
Thus, when inserting data, we use dictionaries.
# Inserting a single document (dictionary).
collection.insert_one({'name': 'Hallvard', 'age': 23})
# Inserting multiple documents (list of dictionaries).
collection.insert_many([
{'name': 'Kristian', 'age': 27},
{'name': 'Ihn Duck', 'age': 15},
])
# Note that an _id field is automatically generated by MongoDB.
InsertManyResult([ObjectId('6750b9fd9ff8af6a87a97d87'), ObjectId('6750b9fd9ff8af6a87a97d88')], acknowledged=True)
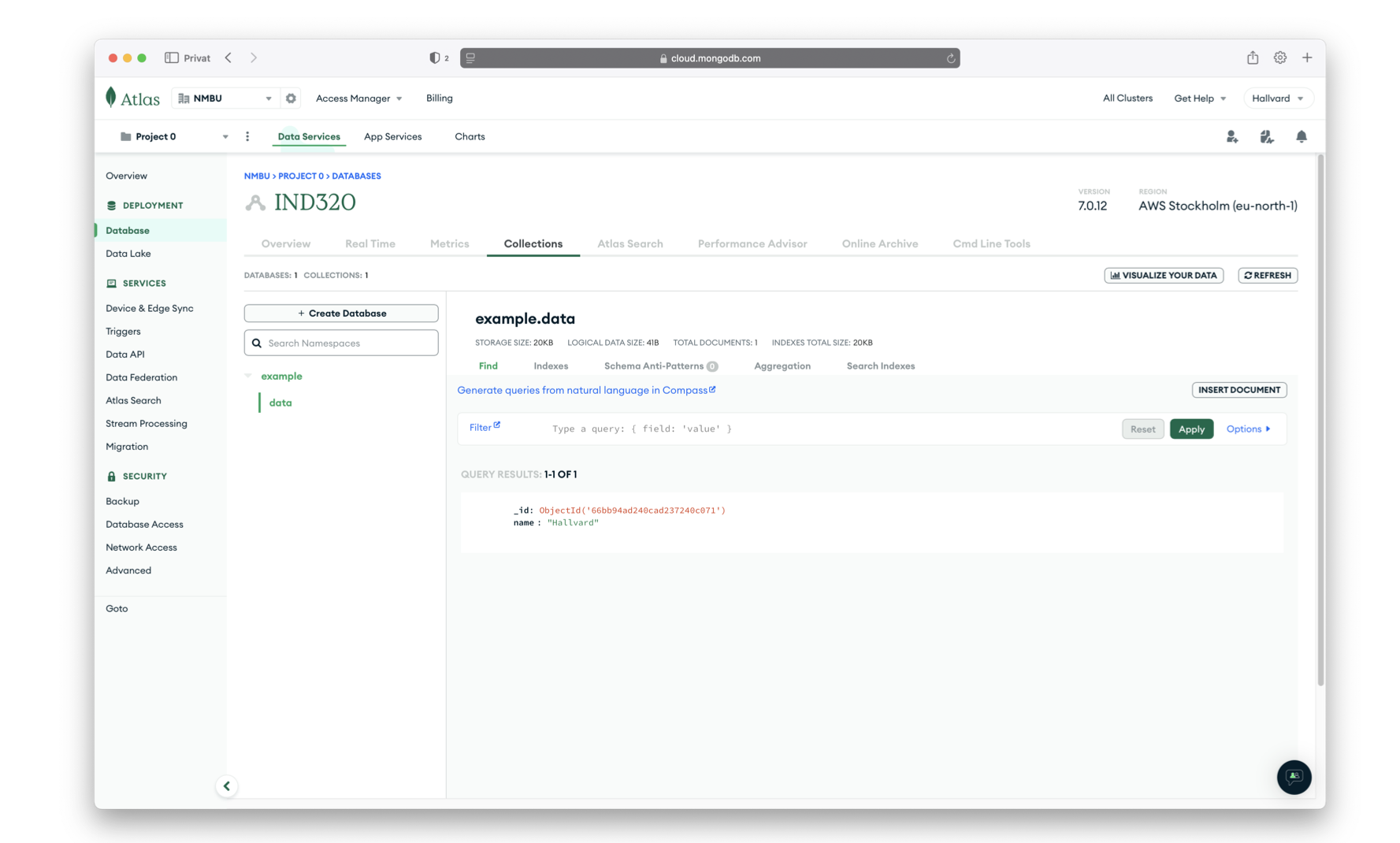
Reading#
# Reading ALL documents from a collection.
# ........................................
documents = collection.find({})
# A cursor is returned.
# The cursor can be iterated over:
for document in documents:
print(document)
# Or directly converted to a list:
documents = list(documents)
# Reading SPECIFIC documents from a collection.
# .............................................
hallvard = collection.find({'name': 'Hallvard'})
for document in hallvard:
print(document)
hallvard = list(hallvard)
{'_id': ObjectId('6750b9fc9ff8af6a87a97d86'), 'name': 'Hallvard', 'age': 23}
{'_id': ObjectId('6750b9fd9ff8af6a87a97d87'), 'name': 'Kristian', 'age': 27}
{'_id': ObjectId('6750b9fd9ff8af6a87a97d88'), 'name': 'Ihn Duck', 'age': 15}
{'_id': ObjectId('6750b9fc9ff8af6a87a97d86'), 'name': 'Hallvard', 'age': 23}
Updating#
Updating documents is done using the update_one and update_many methods.
The first argument is a query that selects the documents to update.
The second argument is a dictionary that specifies the changes.
# Updating a single document.
# ...........................
collection.update_one(
{'name': 'Hallvard'},
{'$set': {'name': 'Hallvard Lavik'}} # Sets the `name` to `Hallvard Lavik`.
)
# Updating multiple documents.
# ............................
collection.update_many(
{},
{'$inc': {'age': 1}} # Increments the `age` of all documents by `1`.
)
UpdateResult({'n': 3, 'electionId': ObjectId('7fffffff0000000000000082'), 'opTime': {'ts': Timestamp(1733343741, 11), 't': 130}, 'nModified': 3, 'ok': 1.0, '$clusterTime': {'clusterTime': Timestamp(1733343741, 11), 'signature': {'hash': b'.7\xea/\xf9d<\x7f\xc5uj\xd6\x90\x9a\x96\x1aE\x95\x83B', 'keyId': 7397429496267145232}}, 'operationTime': Timestamp(1733343741, 11), 'updatedExisting': True}, acknowledged=True)
Aggregating#
Combine multiple operations into a single query.
pipeline = [
{'$match': {'age': {'$gt': 20}}},
{'$group': {'_id': None, 'average_age_over_20': {'$avg': '$age'}}},
]
result = collection.aggregate(pipeline)
result = list(result)
print(result)
[{'_id': None, 'average_age_over_20': 26.0}]
Deleting#
Deleting documents is done using the delete_one and delete_many methods.
# Deleting a single document.
# ...........................
collection.delete_one({'name': 'Ihn Duck'}) # Deletes the document with `name = Ihn Duck`.
# Deleting multiple documents.
# ............................
collection.delete_many({'age': {'$gt': 25}}) # Deletes documents where `age > 25`.
# Deleting all documents.
# .......................
collection.delete_many({})
DeleteResult({'n': 1, 'electionId': ObjectId('7fffffff0000000000000082'), 'opTime': {'ts': Timestamp(1733343741, 14), 't': 130}, 'ok': 1.0, '$clusterTime': {'clusterTime': Timestamp(1733343741, 14), 'signature': {'hash': b'.7\xea/\xf9d<\x7f\xc5uj\xd6\x90\x9a\x96\x1aE\x95\x83B', 'keyId': 7397429496267145232}}, 'operationTime': Timestamp(1733343741, 14)}, acknowledged=True)
See also
Resources